Using jQuery with a CDN For Better Performance (Beginners)
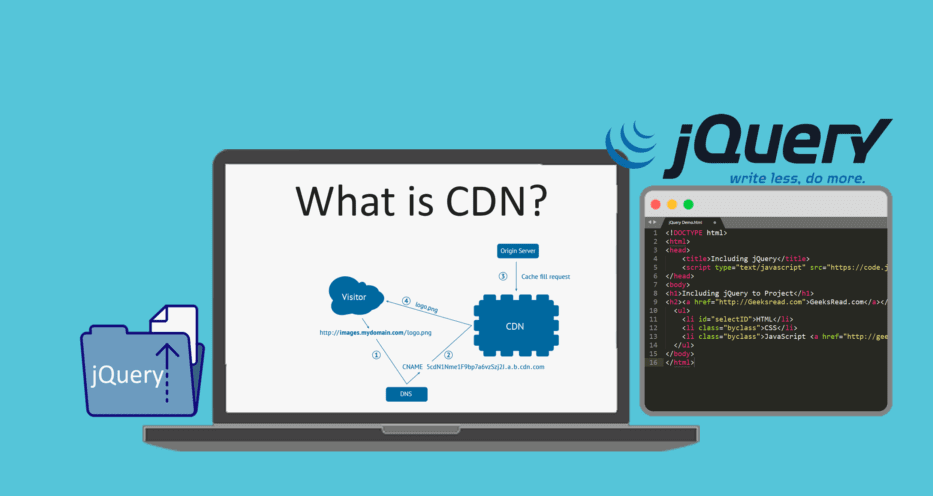
jQuery is perhaps one of the most successful JavaScript libraries out there used to simplify DOM manipulation and create amazing interactivity. If you are a frontend web developer just starting, then you will probably join the jQuery crowd pretty soon or are already using it on your projects. jQuery is not resource-heavy as a library, but it can benefit from CDN caching like any other library out there.
What Is jQuery And What Is It Used For?
jQuery is the single biggest JavaScript library and one that has been around for many years. Nearly every website out there with any type of interactivity or non-static elements has some jQuery in it. The library was created with a “write less, do more” philosophy to simplify frontend coding with JavaScript. You can use jQuery to a lot of things as a frontend developer, among them:
- Document manipulation and traversal- jQuery CDN makes it super-easy to perform all manner of things on documents with few and uncomplicated lines of code.
- jQuery animations and effects- Create smooth frontend animations without having to deal with the internal and complications of vanilla Js.
- Ajax to make server requests
- Manipulate CSS for dynamic styling
- Adds additional utilities to your designs
- Interact with HTML Methods
- Event handling- Handling events such as detecting a mouse hover or click can be a pain in plain JavaScript. However, this can be accomplished with a single line of code as demonstrated below:
Example jQuery Event Handler for Mouseover
("p").mouseover(function (){
$("p").css ("background-color", "red");
});
The above jQuery code will change the element (paragraph) to red whenever a mouse has hovered over it. This same function would probably take several lines of code in Vanilla JavaScript to manipulate the DOM just to get the paragraph to change color.
All in all, jQuery is still a powerful JavaScript library that you may need to use quite frequently on your projects to create all manner of interactive elements and dynamic content. That said, some of its functionality, such as animations, are now mostly implemented using plain HTML5 and CSS animations.
jQuery is a library and therefore needs to be loaded in some way to render your page or other assets that depend on it. This perfectly fine in most cases, but sometimes having to load the library from your server can have a slightly negative impact on your page load speeds hence the need to include jQuery in your projects. Here are some instances where hosting the full jQuery library on your servers could have a negative impact on your website/web app:
You have a limited hosting package- some hosts will provide cheap but restricted hosting packages that may suffer if you have too many assets and dependencies on your website or web application. This will affect your page-load speeds and probably restrict how much you can upload to your server.
JavaScript incompatibilities- This is a rare occurrence nowadays but some hosts may refuse to process your jQuery code due to some incompatibilities within their setup.
How to Use jQuery with a CDN
A content delivery network makes it easier to load libraries like jQuery without having to worry about latency or any other restrictions you might have on your server. It also saves you the hassle of having to download the entire jQuery library, unzipping it and uploading it to the right folder on your web server in order to use it in your projects.
You can add jQuery to your project through a CDN by either referencing it within your head tags and using an open-source jQuery CDN like Google as shown in the code snippet below.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script></head>
Alternatively, you can still have jQuery hosted on your web server and have your website or application loaded through a website CDN. This way, the CDN will use cached versions of your web assets to make it smoother for your visitors to load your website on their devices. Using a CDN for libraries such as jQuery has become so popular and effective at improving user experiences that it has become a best practice in the industry.
Where do I put jQuery CDN in HTML?
The entire jQuery CDN code must be inserted within the head tags in your HTML code for it to be loaded before your elements have rendered. Make sure the CDN is within a script tag as you would normally do when putting an external JavaScript or CSS reference to your HTML.